Six steps to learn about a specific APIs/Libraries and Frameworks with ChatGPT
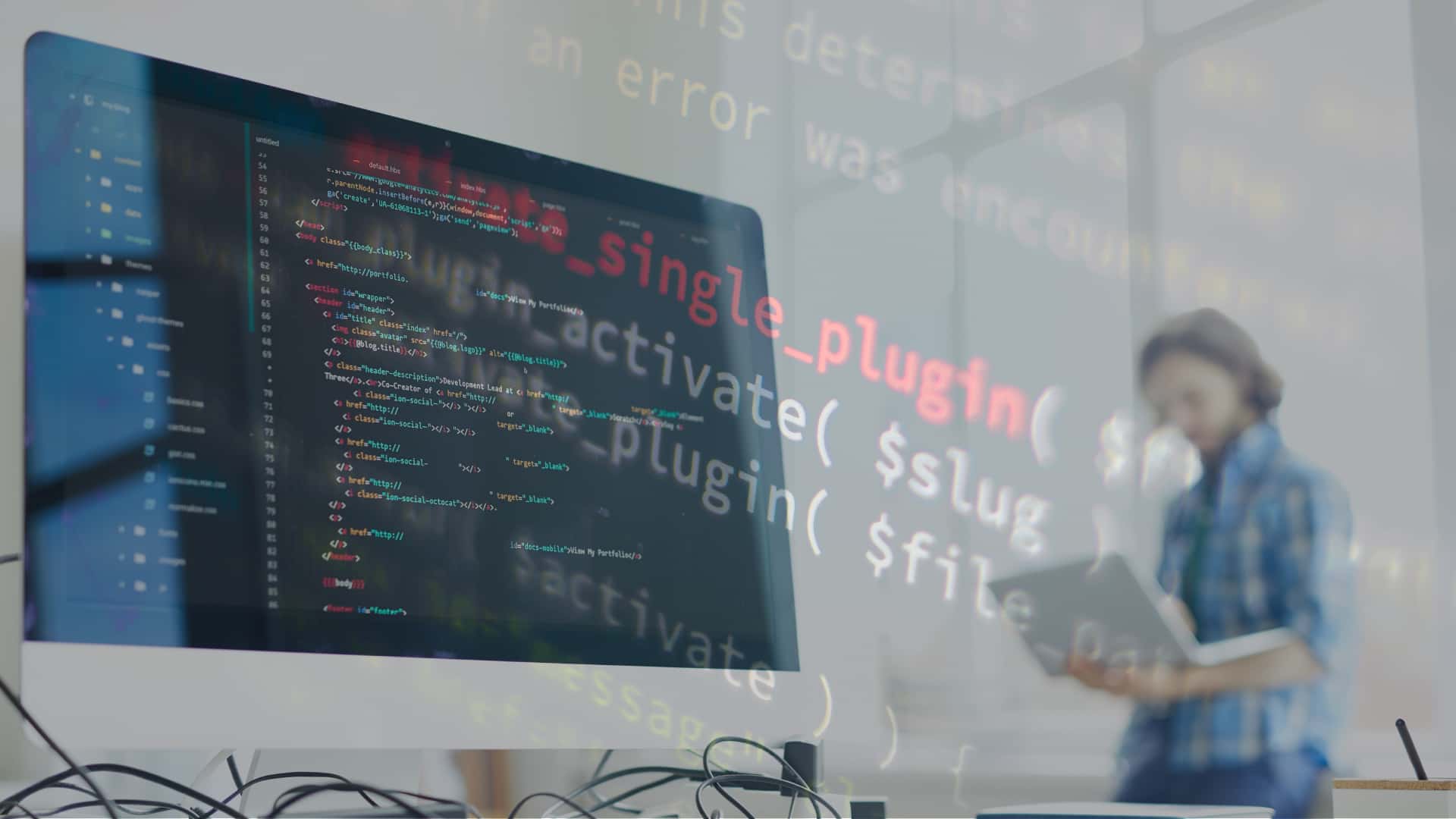
Discover how AI is revolutionizing learning and professional development in the digital age. As technology continues to evolve, it's essential to keep your skills up to date to stay competitive in the job market.
Introduction
As digital technologies continue to expand, more and more people need to learn new skills to stay relevant in the job market. In this context, artificial intelligence (AI) has emerged as a valuable learning and professional development ally.
AI applications are already a reality in our daily lives, making numerous tasks easier. The most talked about technology of the moment is ChatGPT, a chatbot system capable of understanding questions and providing relevant answers on various topics. This tool can assist with simple tasks such as quick searches, discussions on different subjects, and more complex tasks like programming in various programming languages.
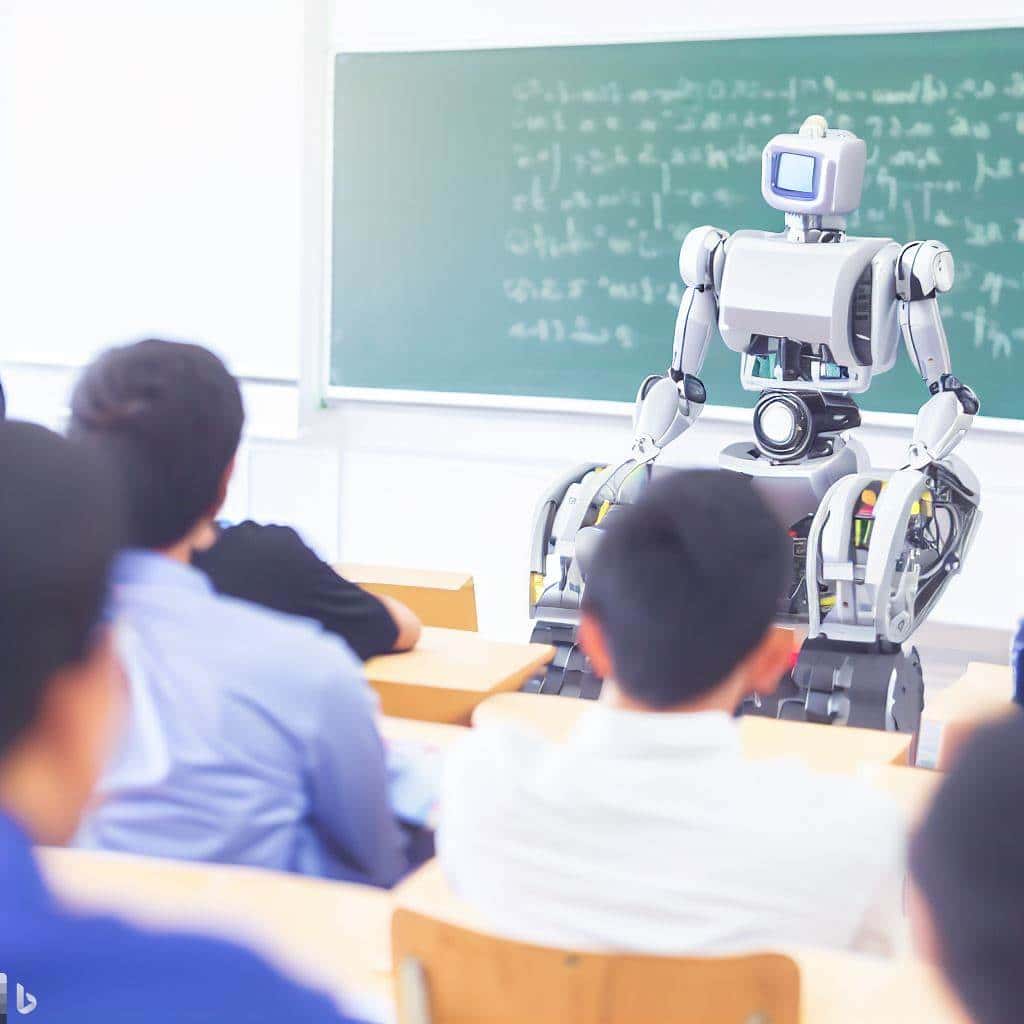
The OpenAi tool ChatGPT has the ability to help developers to learn about any technology, programming language or APIs/Libraries and Frameworks. But asking the right questions is the only requirement to that. Having a list of questions to ask to ChatGPT will help you to evolve from beginner to advanced in that technology.
In order to do that we are going to ask the following questions just changing the keyword to ChatGPT:
- Explain to me the main purpose of the {KEY_WORD} package.
- What are the most common use cases of {KEY_WORD} ?
- Show me a simple TypeORM code example, fully functional.
- Show me a complex use case of {KEY_WORD} code, fully functional.
- List for me the best practices when using {KEY_WORD} .
- What are the anti-patterns to avoid when using {KEY_WORD}?
In our example we'll be using TypeORM as the package we want to learn. Check the following example:
1 - Understanding the main purpose
Question:
Explain to me the main purpose of the TypeOrm package.
Response:
TypeORM is a Node.js package that provides an object-relational mapping (ORM) framework for TypeScript and JavaScript. Its primary purpose is to simplify the interaction with relational databases by allowing developers to map their object-oriented programming language concepts to database objects such as tables, columns, and relationships. This helps developers to write database queries in a more intuitive, object-oriented syntax, rather than having to deal with raw SQL statements.
The key features of TypeORM include:
- Database agnostic: TypeORM supports a variety of relational databases including MySQL, PostgreSQL, SQLite, and Microsoft SQL Server, making it easy to switch between different databases without having to rewrite your database code.
- Entities and relationships: With TypeORM, developers can define entities that map to database tables and relationships between those entities. This allows developers to work with the database in an object-oriented way and to express database relationships as properties on their entity classes.
- Migration support: TypeORM provides tools for managing database migrations, allowing developers to easily update their database schema as their application evolves.
- Query builder: TypeORM provides a powerful query builder that allows developers to construct complex queries in a type-safe and object-oriented way. This makes it easier to build complex database queries without having to write raw SQL statements.
- Data validation: TypeORM provides support for data validation, ensuring that data that is written to the database conforms to defined constraints and business rules.
Overall, TypeORM simplifies the process of interacting with relational databases in Node.js, providing a powerful ORM framework that supports a variety of databases and provides a rich set of features for working with data.
2 - Understanding the most common use cases
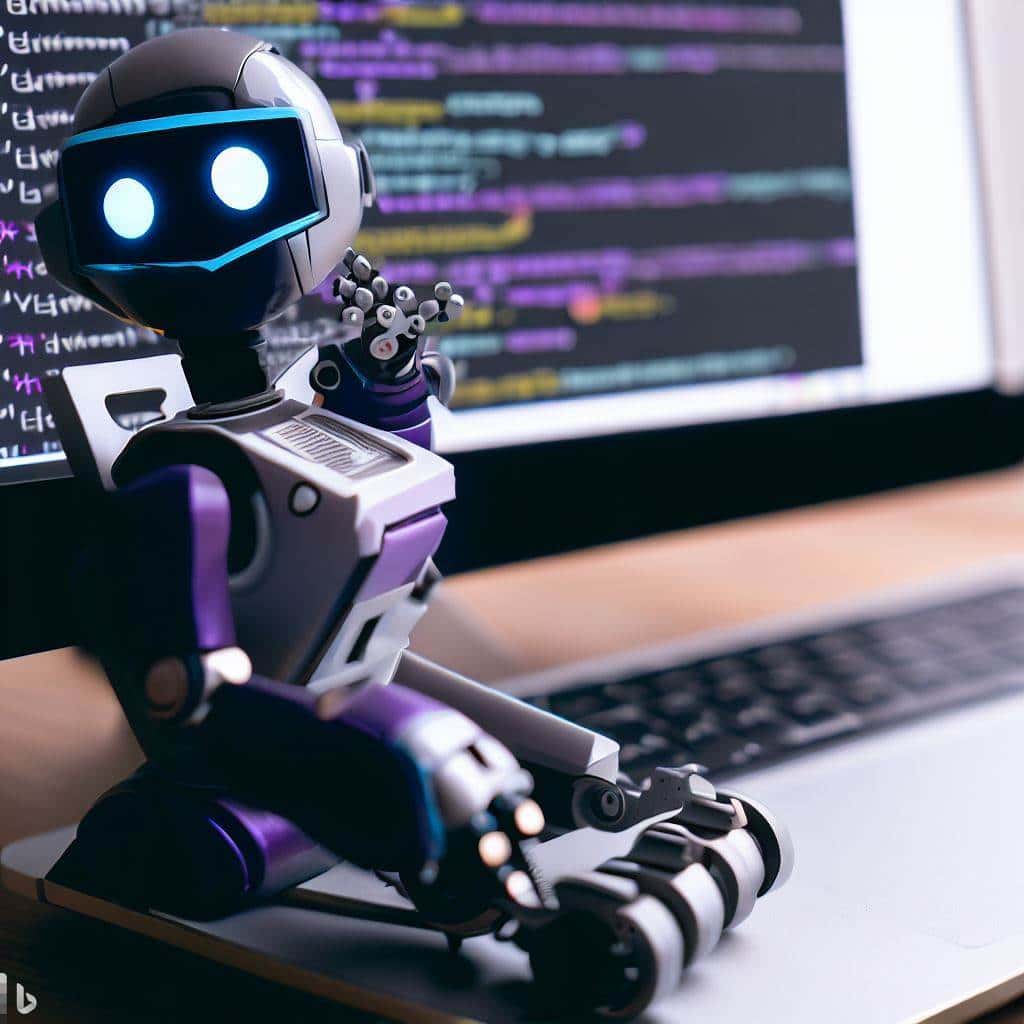
Question:
What are the most common use cases of TypeOrm?
Response:
TypeORM is commonly used in Node.js applications for a variety of use cases, including:
- Building web applications: TypeORM can be used to build web applications that need to store data in a relational database. By using TypeORM, developers can easily map their application data to database tables and build complex queries to retrieve and manipulate data;
- Building APIs: TypeORM can be used to build APIs that need to store and retrieve data from a relational database. With TypeORM, developers can define their database schema using TypeScript or JavaScript classes, and then use the built-in query builder to construct complex queries;
- Building microservices: TypeORM can be used to build microservices that need to store data in a relational database. By using TypeORM, developers can encapsulate their database logic in a single service and provide a clean API for other services to interact with the database;
- Building serverless applications: TypeORM can be used to build serverless applications that need to store data in a relational database. With TypeORM, developers can define their database schema using TypeScript or JavaScript classes, and then use the built-in query builder to construct complex queries, without worrying about server infrastructure;
- Building desktop applications: TypeORM can be used to build desktop applications that need to store data in a relational database. With TypeORM, developers can use the same TypeScript or JavaScript codebase for both their desktop application and their database logic, making it easier to maintain and scale the application.
Overall, TypeORM is a versatile ORM framework that can be used in a variety of applications that need to store data in a relational database.
3 - Building your first use case
Question:
Show me a simple TypeORM code example, fully functional.
Response:
Sure, here's a simple code example of TypeORM that demonstrates how to define an entity, connect to a database, and insert a record into a table.
In this example, we first define a User entity using TypeORM's decorators. The @Entity() decorator marks the class as an entity, and the @PrimaryGeneratedColumn() and @Column() decorators define the primary key and other columns in the table.
Next, we connect to a PostgreSQL database using createConnection(). We specify the database configuration options, including the host, port, username, password, and database name. We also pass an array of entities to TypeORM, which includes the User entity we defined earlier.
Then, we create a new User object, set its properties, and save it to the database using connection.manager.save(). Finally, we close the database connection using connection.close().
Note that this is a very simple example, and there are many more features and options available in TypeORM for working with entities, relationships, queries, and migrations.
4 - Going deeper in your usage
Question:
Show me a complex use case of TypeORM code, fully functional.
Response:
Sure, here's a more complex example that demonstrates how to define multiple entities with relationships, use the query builder to construct complex queries, and use migrations to manage database schema changes.
In this example, we define three entities: User, Post, and Tag, with relationships between them. A User can have many Posts, a Post can have many Tags, and a Post belongs to a single User. We use TypeORM's decorators to define the relationships between the entities.
We then connect to a PostgreSQL database using createConnection(), specifying the database configuration options, including the entities and migrations. We use migrations to manage database schema changes.
We create a new User, Post, and two Tags, and save them to the database using connection.manager.save(). We then use the query builder to construct a complex query that fetches all Posts with their Tags and User authors, using getRepository().createQueryBuilder().
Finally, we close the database connection using connection.close().
5 - Learning the best practices
Question:
List for me the best practices when using TypeOrm.
Response:
Sure! Here are some best practices to follow when using TypeORM:
Define entities with explicit types: When defining your entities, it's important to be explicit about the types of your properties. This makes your code more self-documenting and easier to understand.
Use the query builder: TypeORM provides a powerful query builder that can help you construct complex queries without writing raw SQL. Use it instead of raw SQL queries to keep your code more organized and maintainable.
Use migrations: Use TypeORM's migrations feature to manage changes to your database schema over time. This will help ensure that your database schema stays in sync with your code.
Use transactions: Use transactions to ensure data integrity and consistency. Transactions help ensure that a set of database operations either complete successfully or are rolled back if there's an error.
Use eager loading: Use TypeORM's eager loading feature to specify which related entities to load with your primary entities. This can help reduce the number of queries your application needs to make to the database.
Use the repository pattern: Use the repository pattern to abstract away database access from the rest of your code. This makes your code more modular and easier to test.
Use database pooling: Use database pooling to improve performance and reduce the overhead of opening and closing database connections.
Use a connection manager: Use a connection manager to manage your database connections. This will help ensure that you don't create too many connections and will allow you to reuse existing connections where possible.
Handle errors gracefully: Be sure to handle errors gracefully by logging them and returning meaningful error messages to your users. This will help your application be more robust and user-friendly.
Keep your dependencies up-to-date: Keep your dependencies up-to-date to ensure that you're using the latest features and bug fixes. Also, be sure to check for any security vulnerabilities in your dependencies and update them as needed.
6 - Learning the anti-patterns
Question:
What are the anti-patterns to avoid when using TypeORM?

Response:
Here are some anti-patterns to avoid when using TypeORM:
Not defining entity relationships: Failing to define relationships between entities can lead to inefficient queries and other issues down the line.
Using raw SQL: While TypeORM does allow for executing raw SQL queries, it's best to avoid them whenever possible. Raw SQL can be error-prone, harder to maintain, and can make your code more vulnerable to SQL injection attacks.
Not using transactions: Failing to use transactions when modifying data can lead to data inconsistencies and corruption in your database.
Not using eager loading: Failing to use eager loading can lead to the N+1 problem, where your application makes many more database queries than necessary, leading to performance issues.
Not using migrations: Not using migrations can lead to inconsistencies between your database schema and your code, making it harder to maintain and debug.
Not using database pooling: Not using database pooling can lead to slower performance and increased overhead for opening and closing database connections.
Not handling errors: Failing to handle errors can make your application less robust and harder to debug.
Not using the repository pattern: Failing to use the repository pattern can lead to code that's tightly coupled to the database, making it harder to test and maintain.
Creating too many connections: Creating too many connections to the database can lead to performance issues and can cause your application to run out of resources.
Not optimizing queries: Not optimizing queries can lead to inefficient database access, resulting in slower performance and increased resource usage.
7 - Going Deeper
These six steps presented are only the tip of the iceberg. For instance, the user can use each of the outputs of ChetGPT to go even deeper into the learning process. Here are some other possibilities the user may want to test:
7.1 Get examples of the best practices proposed
Question:
Show me a code example of the typeORM best practice you suggested to me: Define entities with explicit types: When defining your entities, it's important to be explicit about the types of your properties. This makes your code more self-documenting and easier to understand.
Response:
Sure! Here's an example of defining entities with explicit types using TypeScript:
In this example, we define a User entity with four columns: id, name, email, and isActive. Each column is explicitly defined with a specific type using the type property. We also specify the length property for the name and email columns to ensure they have a maximum length. Finally, we set the default value for the isActive column to true.
By being explicit about the types of each column, we can make our code more self-documenting and easier to understand. This is especially important when working on a large codebase or with a team, as it can help prevent bugs and make the codebase more maintainable.
7.2 Get examples of the antipatterns to avoid:
Question:
Show me now an example of code using TypeORM that has this anti-pattern and another one showing how to avoid the following anti-pattern: Using raw SQL: While TypeORM does allow for executing raw SQL queries, it's best to avoid them whenever possible. Raw SQL can be error-prone, harder to maintain, and can make your code more vulnerable to SQL injection attacks.
Response:
Sure! Here's an example of code that uses the anti-pattern of using raw SQL in TypeORM:
In this example, we use the query method on the connection object to execute a raw SQL query. The query is written as a string and is not type-checked, making it more error-prone and harder to maintain. Additionally, this code is vulnerable to SQL injection attacks.
Here's an example of how to avoid using raw SQL in TypeORM by using the QueryBuilder API instead:
In this example, we use the createQueryBuilder method to create a query builder object and chain a series of methods to build up our query. The query is type-checked and is less error-prone and easier to maintain than the raw SQL version. Additionally, this code is not vulnerable to SQL injection attacks.
Conclusion
In conclusion, the use of artificial intelligence in learning new technologies provides several advantages. It can create personalized learning experiences, offer real-time feedback, and improve the efficiency and effectiveness of learning programs. AI can also help individuals to acquire new skills quickly and with greater accuracy. As AI technology continues to advance, it will become an increasingly valuable tool for individuals looking to stay competitive in their respective fields. The benefits of AI-assisted learning are clear, and this technology will play a vital role in shaping the future of education and training. From beginner to advanced, ChatGPT is the perfect tool for mastering any technology.
Try it out now at https://chat.openai.com/chat